Organizing View Logics in Laravel with View Composers
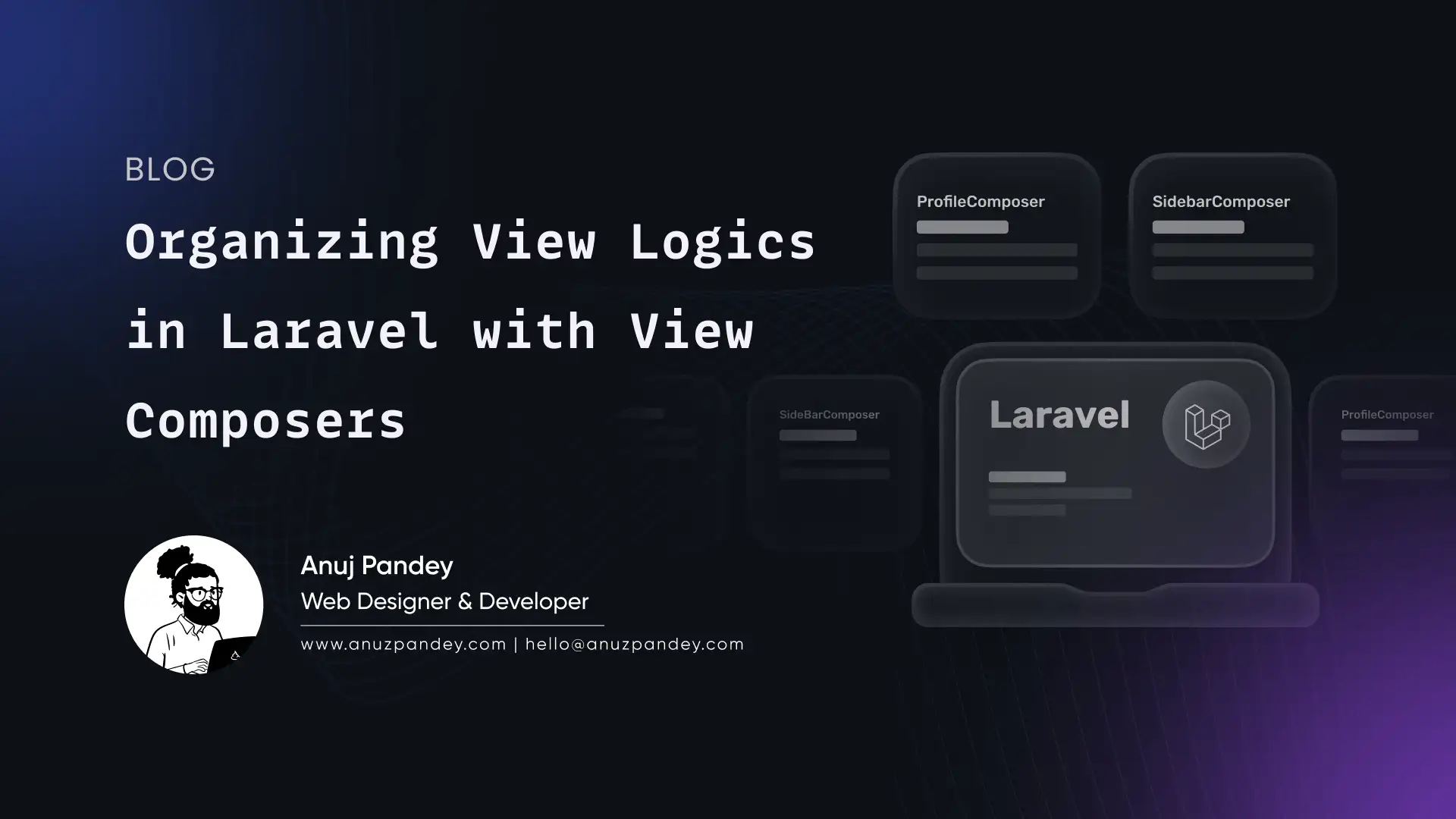
When building Laravel applications, you might often find yourself passing the same data to multiple views. This not only leads to repetitive code in your controllers but also increases the chances of data inconsistencies. Laravel’s View Composers offer a neat solution by helping you manage view-specific logic more efficiently.
What Are View Composers?
View composers are pieces of code that prepare data for views. They’re like middlemen that inject data into views whenever they’re rendered. With View Composers, you can centralize your view logic, making your controllers cleaner and your application more organized.
Creating a Basic View Composer
You typically register a view composer in a service provider, like the AppServiceProvider. Let’s look at a simple example:
Next, define the ProfileComposer class that will handle the data preparation:
Everytime the footer-links view is rendered, the compose method runs, passing the footerLinks data to the view.
Using Closures for Simplicity
If your view composer is straightforward, you can use closures instead of creating a separate class:
Handling Multiple Views with Wildcards
You can use wildcards to attach a composer to a group of views:
In this example, the AccountComposer will be applied to all views under the accounts folder.
Real-World Example: Dynamic Navigation Menus
Let’s see a practical use case where we want to populate a navigation menu with dynamic data.
- Create a NavigationMenuComposer class:
- Register the Composer in AppServiceProvider:
- Update Your navigation-menu.blade.php template:
In this example, the NavigationMenuComposer automatically provides data for popular categories and recent categories to the view, keeping our navigation menu consistent and our controllers clutter-free.
Why Use View Composers?
- Centralized Logic: All view-specific logic stays in one place, making it easier to manage.
- Cleaner Controllers: Move data preparation out of your controllers, keeping them focused on business logic.
- Consistency: Avoid inconsistencies by defining view data in a single composer, ensuring your views always get the correct data.
Conclusion
Whether you’re building complex dashboards, dynamic sidebars, or any feature that relies on shared view data, Laravel’s view composers are a powerful tool to keep your code organized and maintainable.
Your feedback is valuable to me, so if you'd like to see more content like this in the future, please don't hesitate to let me know.
Keep coding, keep exploring, and keep inspiring. 🐼